开发来电显示及录音程序的C#范例 |
chen在2010/3/7发表,被浏览9126次
此文章共有 2 页
1
2
|
功能: (1)来电显示,并把来电记录保存到数据库; (2)可设置状态,让来电显示管理器自动录音,并把声音文件名保存到数据库。 源程序JDTest.rar:(点击下载) 控件包JDComPort.rar(点击下载 ) 详情请看:来电管理设备的ActiveX控件(OCX)开发文档 相关文档:C#来电显示管理器开发示例程序 源代码如下:
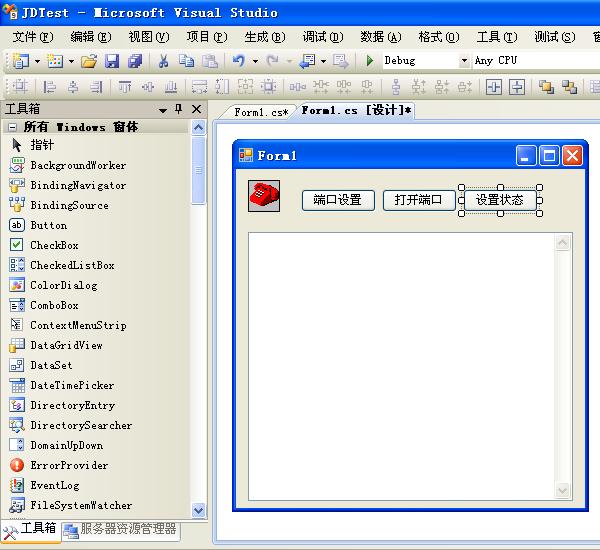
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; using System.IO; using System.Runtime.InteropServices; using System.Data.OleDb; namespace JDTest { public partial class Form1 : Form { const byte DEVCOUNT = 4; //1个来电盒最多可连接4条电话线 public string dbFileName, wavPath; public TPressKey[] aPK = new TPressKey[DEVCOUNT]; //记录每条电话线的状态 public void InitAPK() { for (byte i = 0; i < DEVCOUNT; i++) { if (aPK[i] == null) aPK[i] = new TPressKey(); aPK[i].initPressKey(); } } public TPressKey FindPKey(string devid) { for (byte i = 0; i < DEVCOUNT; i++) if (aPK[i].devid == devid) return aPK[i]; for (byte i = 0; i < DEVCOUNT; i++) if (aPK[i].devid == "") { aPK[i].devid = devid; aPK[i].t = DateTime.Now; return aPK[i]; } return aPK[0]; } public TPressKey FindDialPK() { for (byte i = 0; i < DEVCOUNT; i++) if (aPK[i].b == JDStatus.Dial) return aPK[i]; return aPK[0]; } //-------------------------------------------------------------------- public Form1() { InitializeComponent(); dbFileName = System.Windows.Forms.Application.StartupPath + "\\db1.mdb"; wavPath = System.Windows.Forms.Application.StartupPath + "\\Rec\\"; IniFile ini = new IniFile("JD2000ocx.ini"); if (ini.IniExistFile()) wavPath = ini.IniReadValue("System", "WavFilePath", wavPath); } private void button1_Click(object sender, EventArgs e) { //设置来电管理器连接端口,第一次连接时使用 axJDComponent1.SetupPorts(); } private void button2_Click(object sender, EventArgs e) { axJDComponent1.Open(); } private void button3_Click(object sender, EventArgs e) { //设置录音方式 axJDComponent1.SetSate(); } private void axJDComponent1_OnOpen(object sender, EventArgs e) { textBox1.Text = "JD Open\r\n"; InitAPK(); } private void axJDComponent1_OnClose(object sender, EventArgs e) { textBox1.Text += "JD Close\r\n"; } private string connectionString() { return "Provider=Microsoft.Jet.OLEDB.4.0; User ID=Admin; Password=; Data Source=" + dbFileName; } private string appendRecord(string devid, string s, DateTime t) { string id = DateTime.Now.ToString("yyMMddhhmmssfff"); OleDbConnection conn = new OleDbConnection(connectionString()); conn.Open(); OleDbCommand cmd = new OleDbCommand("Insert Into Tele (id, devid, s, t) Values('" + id + "','" + devid + "','" + s + "',CDate('" + t.ToString("yyyy-MM-dd hh:mm:ss") + "'))", conn); cmd.ExecuteNonQuery(); conn.Close(); return id; } private void updateRecord(TPressKey pk) { OleDbConnection conn = new OleDbConnection(connectionString()); conn.Open(); string str = "Update Tele Set k=" + ((int)pk.b).ToString(); if (File.Exists(wavPath + pk.wavfile)) str += ", wav='" + pk.wavfile + "'"; str += " Where id='" + pk.id + "'"; if (pk.b==JDStatus.Talk) str += " and devid='" + pk.devid + "'"; //textBox1.AppendText(str + "\r\n"); OleDbCommand cmd = new OleDbCommand(str, conn); cmd.ExecuteNonQuery(); conn.Close(); //textBox1.AppendText("Update Success!\r\n"); } private void axJDComponent1_OnRead(object sender, AxJDCompPort.IJDComponentEvents_OnReadEvent e) { //当有电话打入时 DateTime t = DateTime.FromOADate(e.t); TPressKey pk; if (e.devid == "D") pk = FindDialPK(); else pk = FindPKey(e.devid); pk.wavfile = e.waveFile; pk.id = appendRecord(e.devid, e.s, t); textBox1.AppendText(e.devid + " " + e.s + " " + t.ToString("MM-dd hh:mm:ss") + "\r\n"); //textBox1.AppendText(pk.devid+" : "+pk.wavfile + "\r\n"); } private void axJDComponent1_OnKeyPress(object sender, AxJDCompPort.IJDComponentEvents_OnKeyPressEvent e) { textBox1.AppendText(e.devid + " " + e.key + "\r\n"); TPressKey pk = FindPKey(e.devid); switch (e.key) { case "R": //响铃 pk.setStatus(JDStatus.Ring); break; case "T": //提取话筒 if ((pk.b == JDStatus.Ring) & (pk.keyInterval() < 3000)) //3秒内 { if (pk.id != "") { textBox1.AppendText(e.devid + " " + "接听来电" + "\r\n"); updateRecord(pk); } pk.setStatus(JDStatus.Talk); //来电已接听 } else { pk.setStatus(JDStatus.Dial); } break; case "H": //放下话筒 if (File.Exists(wavPath + pk.wavfile)) { textBox1.AppendText("录音:" + pk.wavfile + "\r\n"); updateRecord(pk); } pk.id = ""; pk.wavfile = ""; pk.setStatus(JDStatus.Idel); break; } } //===================================================================== public class IniFile { //文件INI名称 public string Path; ////声明读写INI文件的API函数 [DllImport("kernel32")] private static extern long WritePrivateProfileString(string section, string key, string val, string filePath); [DllImport("kernel32")] private static extern int GetPrivateProfileString(string section, string key, string def, StringBuilder retVal, int size, string filePath); [DllImport("kernel32")] private static extern void GetWindowsDirectory(StringBuilder WinDir, int count); //类的构造函数,传递INI文件名 public IniFile(string inipath) { if ((inipath.Substring(2,1)==":")||(inipath.StartsWith("\\\\"))) Path = inipath; else Path = getWindowsDir() + "\\" + inipath; } public string getWindowsDir() { StringBuilder temp = new StringBuilder(255); GetWindowsDirectory(temp, 255); return temp.ToString(); }
|
|
|
|